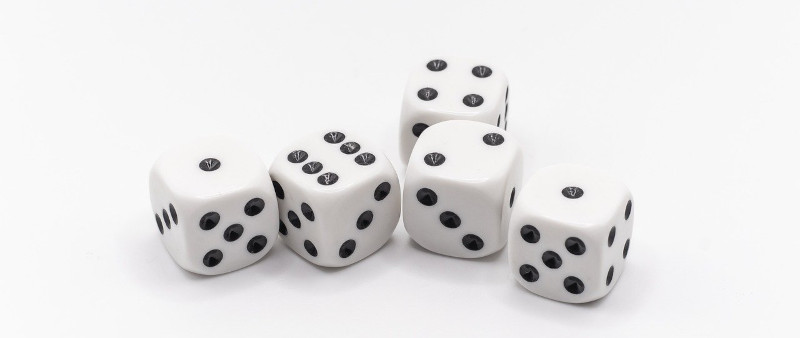
The usefulness of randomness is not limited to just colors.
Random numbers are used so often in computer science that nearly every programming language provides some function for generating ‘pseudo-random‘ numbers.
They are called pseudo-random because most implementations cannot actually generate truly random numbers but instead rely on some nondeterministic source, such as the current time, to compute a “random” number.
print(math.random())
‘math‘ is a built-in Lua library that provides additional math functionality in addition to the ‘random‘ function. We will learn more about libraries later.
If you do not give the function an argument, it will return a random number between 0 and 1.
You can generate integers in some range by passing in one or two integer arguments.
print(math.random(10))
print(math.random(-10, 10))
With a single integer argument, the range will be from 1 up to and including that integer—so in this case, 10.
The single argument version needs to be a positive integer, so if you need a negative number or a range outside of 1 to x, you can provide the function with a number range.
The second statement in the example will print out a number from -10 to 10.
Going back to our part color, we can use this to create a random color similar to BrickColor, albeit with a wider color palette:
local part = game.Workspace.Part
local red = math.random()
local green = math.random()
local blue = math.random()
part.Color = Color3.new(red, green, blue)
print(red, green, blue)