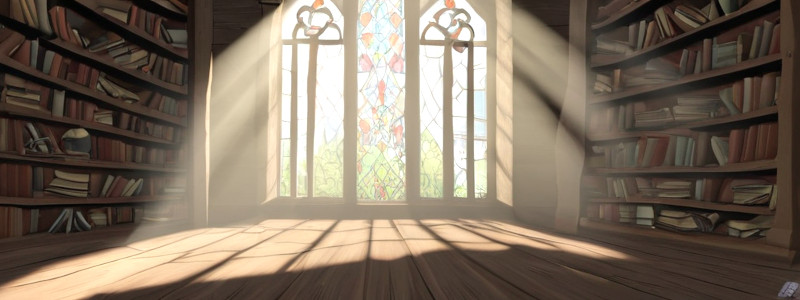
Libraries are a collection of pre-written code for performing specific tasks. Most languages include “standard libraries” to supplement the base language.
Lua and Roblox also include their own libraries to aid in development on the platform.
OS Library
os.time
Apparently timekeeping is something that humans are really into. So much so that we invented quite a number of different ways to track time.
The ‘os.time’ function is similar to the tick function, however, its time is synced to UTC and not the local device and does not return fractional seconds.
print(tick()) --1715253979.3176708
print(os.time()) --1715251979
But more importantly, os.time can also accept a table argument that specifies a date. Then the returned result will be the time elapsed (in seconds) since Unix epoch and that date.
local date = {["year"]=2020,
["month"] = 12,
["day"] = 25
}
print(os.time(date)) --1608897600
os.date
The os.date function returns a string formatted date. If no argument is given, returns the current date in the format:
print(os.date()) --Tue Apr 10 12:55:49 2024
A ‘format specifier’ can be passed to the function, specifying how you want the date to be formatted.
This is a string made up of a cryptic syntax borrowed from the C programming language, each specifier beginning with the ‘%’ symbol.
print(os.date("%m-%d-%Y")) --04-19-2024
print(os.date("%d-%m-%Y")) --19-04-2024
print(os.date("%Y/%m/%d")) --2024/04/19
‘d’ for day, ‘m’ for month, ‘y’ or ‘Y’ for year, etc. The specifier acts as a placeholder that gets replaced by the proper date-time value and then returned from the function.
A second argument specifying the Unix time can also be passed to the function. The returned string will then be synced for that date.
local date = {["year"]=2021,
["month"] = 2,
["day"] = 29
}
local ts = os.time(date)
print(os.date("My birthday is %B %d, %Y", ts))
--My birthday is March 01, 2021
If the specifier is ‘*t’ or ‘!*t’, a table containing key-value pairs for the date is returned rather than the date.
local date = {["year"]=2021,
["month"] = 2,
["day"] = 29
}
local ts = os.time(date)
print(os.date("!*t", ts))
--{ ["day"] = 1,
-- ["hour"] = 12,
-- ["isdst"] = false,
-- ["min"] = 0,
-- ["month"] = 3,
-- ["sec"] = 0,
-- ["wday"] = 2,
-- ["yday"] = 60,
-- ["year"] = 2021 }
The os.date function is included as a part of the Lua standard library. The DateTime class is a Roblox specific class that features extended date-time related functionality.
os.clock
I would much rather write an entire section on why you should NOT concern yourself with the performance of your code at this stage. But you will only completely ignore that and then go chasing performance anyway.
And the developers have indicated (back in 2020) that they intend to deprecate the ‘tick’ function.
It’s good to have a backup plan.
os.clock is a high precision timer, similar to tick, making it useful for benchmarking and timing.
local startTime = os.clock()
local number = 0
for i = 1, 1000000 do
number = number + math.random()
end
local et = os.clock() - startTime
print("Elapsed time:", et)
Math Library
We won’t go over all of the math functions since most of them are self-explanatory and the others, we’ll go over when we cross that bridge.
I’ll only draw attention to few so that you are at least aware of what they do.
randomseed
This sets the “seed” for the random number generator.
Because the random algorithm cannot generate a truly random number, it uses an external source to initialize that randomness. This seed can be from an indeterminate source such as the clock time or even a dedicated hardware generator.
The randomseed function allows you to manually set the seed.
math.randomseed(3.14)
print(math.random())
--0.18063660386662542
math.randomseed(3.14)
print(math.random())
--0.18063660386662542
math.randomseed(3.14)
print(math.random())
--0.18063660386662542
The random sequence is always the same so for some unvarying seed, the output will also be the same.
ceil, floor, round
The ‘ceil’ function returns an integer that is larger or equal to the input. On the other hand, the ‘floor’ function returns the largest integer that is less than or equal to the input.
print(math.ceil(3)) -- 3
print(math.floor(3)) -- 3
print(math.ceil(3.01)) -- 4
print(math.floor(3.99)) -- 3
print(math.ceil(-3.01)) -- -3
print(math.floor(-3.99)) -- -4
Neither of these functions perform any rounding so beware of that. Use the ’round’ function instead if your application requires rounding.
print(math.round(0.5)) --1
print(math.round(0.4)) --0
print(math.round(-0.4)) --0
print(math.round(-0.5)) -- -1
huge
This is a property from the math library and represents infinity.
print(math.huge)
print(-math.huge)
--inf
-- -inf
print(math.huge - math.huge)
-- nan
Any value that can be represented by the number data type will always be less than math.huge and greater than -math.huge.