All functions “return” whether or not it is explicitly defined. Similar to the break keyword, if an earlier return is required, the return keyword will cause the function to stop execution and return from the function.
local function doSomething(argument)
if not argument then
print("No argument given.")
return
end
print("Received argument.")
return
end
doSomething() --Output: No argument given.
doSomething(1) --Output: Received argument.
Often we also want the function to return its result and we can do so via the return keyword. Any data appended to the return will be passed to the parent scope when the function exits.
local function getRandomList(numberOfElements)
local tempList = {}
for i = 1, numberOfElements do
tempList[i] = math.random()
end
return tempList
end
randomNumbersList = getRandomList(10)
print(randomNumbersList)
Returns are not limited to just returning a single result. To return multiple results, separate each using a comma.
local function getSumAndProduct(numberList)
local sum = 0
local product = 1
for _, number in ipairs(numberList) do
sum = sum + number
product = product * number
end
return sum, product
end
local list = {1, 2, 4, 8, 16}
local sumResults, productResults = getSumAndProduct(list)
print(sumResults) --Output: 31
print(productResults) --Output: 1024
Variables also need to be in place to “catch” the result as we did in line 14. Separate these using commas as well.
The Stack
The stack is a large region of memory reserved for temporary data. When the thread invokes a function, that function is given a stack frame, which is then “pushed” onto the stack. Among other things, the stack frame contains local variables and data that the function needs for its task.
Stack frames are organized like a stack of paper in that the topmost frame contains the data for that thread’s most recent function call. Then each subsequent function call is stacked atop that.
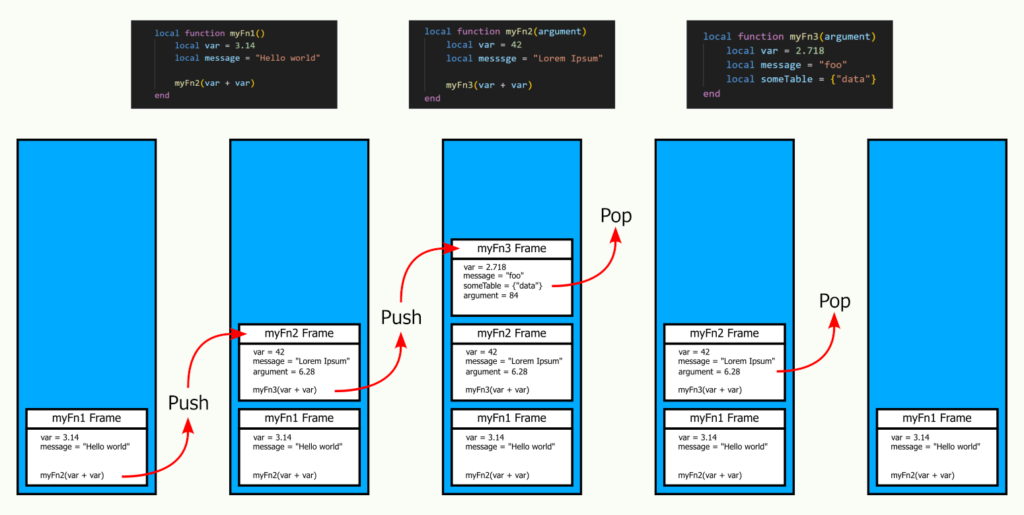
After the function returns, the stack frame is “popped” and that segment of memory is returned to the stack. The calling function cannot return until the function it invoked returns, making stacks operate in a “last in, first out” (LIFO) manner.
Because the memory is returned, the data in that frame is lost if not returned or stored elsewhere.