We’ve used several functions but thus far, we’ve only treated them as a sort of machine that takes in an input, runs some mystery routine, and then outputs a result.
So what are functions?
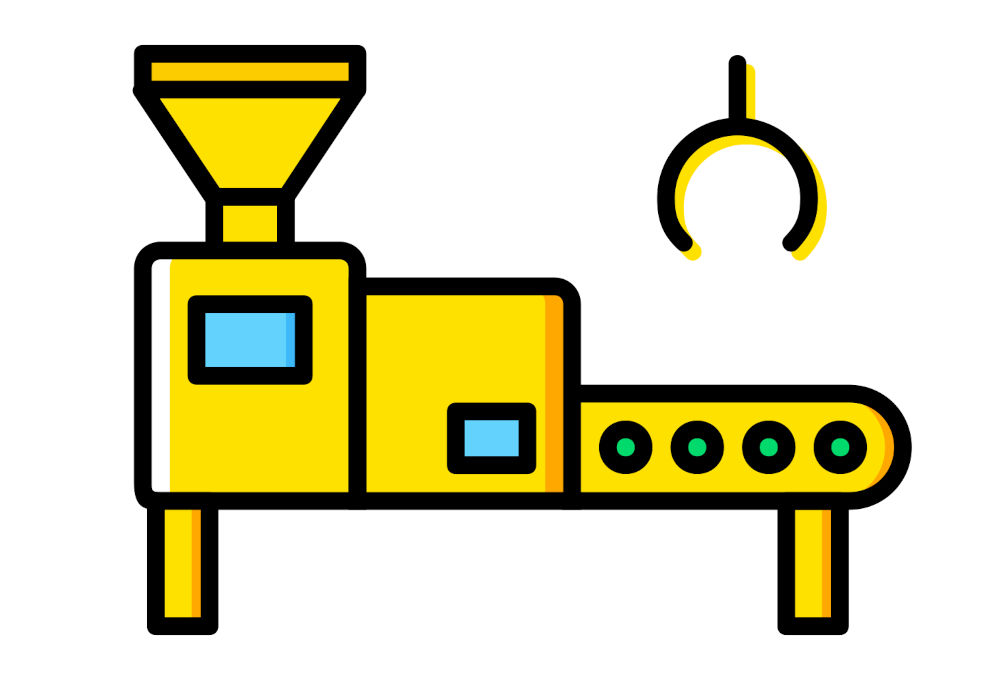
A function is a pre-defined and self-contained block of that can be invoked from another section of code. This makes that block of code “reusable” such that you Don’t Repeat Yourself having to implement code to perform some similar or identical task numerous times.
This concept of collecting all of the relevant components for a task and wrapping them behind some container and then providing only an interface for the functionality is called abstraction.
Let’s say you wanted to create a part at some position. One way to do it is to just copy and paste the block of code and modify the different parameters.
local part1 = Instance.new("Part")
part1.Position = Vector3.new(3.14, 5, 42)
part1.BrickColor = BrickColor.new("Lime green")
part1.Anchored = true
part1.Shape = Enum.PartType.Cylinder
part1.Parent = game.Workspace
local part2 = Instance.new("Part")
part2.Position = Vector3.new(6.28, 5, 84)
part2.BrickColor = BrickColor.new("Hot pink")
part2.Anchored = true
part2.Shape = Enum.PartType.Cylinder
part2.Parent = game.Workspace
And while this works perfectly fine, can you already see that much of this code is duplicate. If you had to add or change some property for all the parts, you’d have to go back and modify it wherever this block shows up.
The variable solved a similar problem for singular references to data. Functions expand on that by enclosing and referencing blocks of code.
Functions are defined using the function keyword before a variable identifier followed by the parenthesis notation.
local function sayHello()
print("Hello world!")
end
Of course, this only defines the function but it does not call the function. You can call the function in the same manner that we’ve been doing.
local function sayHello()
print("Hello world!")
end
sayHello() --Output: Hello world!
sayHello() --Output: Hello world