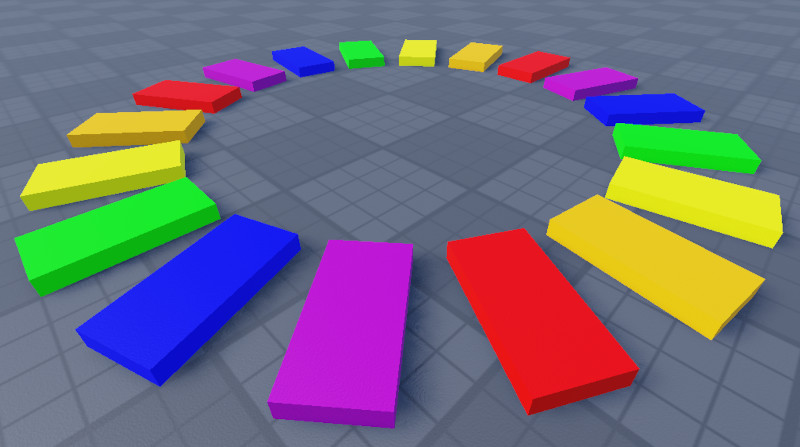
If you look at the properties for a part, you will see that the part has both a ‘BrickColor‘ and a ‘Color‘ property. But which do you use?
Just like data needs a type to have any meaning, object properties also need its values formatted as the correct data type. The Name attribute is a string datatype, for instance.
Both BrickColor and Color3 do the same thing: set the color of a part. Each property requires the color encoded using a different data type and the other property will automatically update to reflect the change.
The Color3 datatype covers the full spectrum of 16,777,216 colors and the combinations are reflected in its constructor arguments. But most of us are bad at remembering so many numbers. We are slightly better at remembering names.
BrickColor
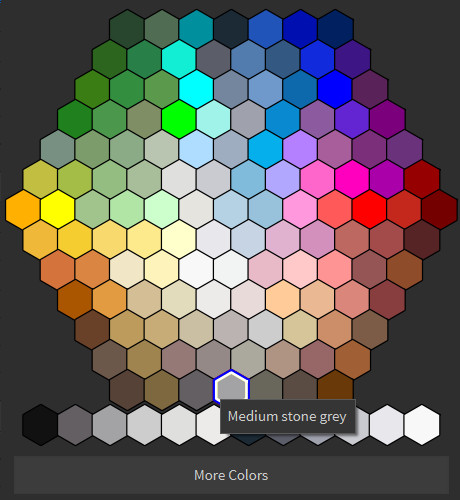
BrickColor is a preset list of common colors that can be constructed using a name rather than some number combination. Currently, there are 208 BrickColors as opposed to the 16 million+ Color3 colors.
You can view the complete list of preset color names on the API reference.
Like you saw previously, BrickColor uses a ‘new‘ constructor and returns the configured datatype required to set that property.
local part = game.Workspace.Part
part.BrickColor = BrickColor.new("Lime green")
This will turn the part lime green.
The new constructor can also accept a single integer value that represents the preset color. These values are also found on the API.
local part = game.Workspace.Part
part.BrickColor = BrickColor.new(1032)
Another functionality BrickColor provides is a ‘Random‘ constructor that returns a random color type. Unlike the new constructor, this does not take a constructor argument.
local part = game.Workspace.Part
part.BrickColor = BrickColor.Random()
Each time you run the code, the part will be assigned a random color.
Color3
If you desire a color not available under BrickColor, you can create your own with the Color3 datatype. This provides for a much wider color palette.
local part = game.Workspace.Part
part.Color = Color3.new(0, 1, 1)
The new constructor requires three arguments with values from 0 up to and including 1 to represent Red, Green, and Blue.