You see, computers are not very smart (I hope this does not come back to bite me).
When a computer encounters some line of code, it also needs instructions on how to interpret that code.
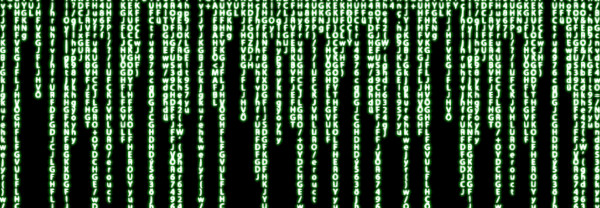
We saw that print is a pre-defined function. But ‘Hello world!‘ is not a function.
It’s just a series of 1’s and 0’s stored in memory. This is not very useful because Lua does not know what to do with it.
Part of the reason for a language’s syntax and semantics is to give context to some set of data.
This ‘data type‘ is a collection of data that has certain attributes associated with it. It tells Lua how to interpret the data and in turn what instructions are available for working with it.
Strings
Whenever single or double quotes are placed around some portion of text, it tells the interpreter to treat it literally as text and not instructions.
This is so that Lua does not attempt to, say, run it as a function.
print("print()")
Strings can include almost any number or character.
In the example above, the text matches the ‘print()‘ statement, but because it is enclosed within quotes, Lua will interpret that text as a string and not as a function or statement.
Numbers
In computer science, numbers usually come in two different types and this affects how they are implemented and stored in memory.
Simplest is the integer. This is any number that does not contain a decimal or fractional component. So 1, -30, 42 are all integers. 1.1, 3.14, -10.5 are not integers.
And then the float. Short for ‘floating point number,’ this is any number that has a decimal component. So -0.25, 3.14, 99.99 are all floats.
For Lua 5.1, which Roblox scripting is based on, there is not a distinction between the two and so integers and floats are grouped together simply as the ‘number‘ data type.
This is a just a peculiarity of the language and will have little consequence for most projects you work on.
Unlike the string datatype, numbers are not enclosed in quotes:
print(3.14) --Output: 3.14
Boolean
One of the things that make computers are useful is their ability to make decisions. But at it’s most basic, those decisions are made based on whether some value is true or false. 1 or 0.
For most other languages, ‘true‘ and ‘false‘ can also be represented by 1 or 0.
But Lua (5.1) is not most other languages. Remember the part about Lua integers being implemented as floats? This means that in memory, 1 and a 0 are not stored as 1 and or a 0 but instead, a long string of 1’s and 0’s of the float data type that is equivalent to 1.0 or 0.0.
The Boolean data type is made up of exactly two values: ‘true’ and ‘false’ and represent 1 and 0 in the traditional sense.
Nil
Sometimes, rather than representing data, it is useful to represent the absence of any data at all. This data type consists only of the value ‘nil.’
Lua has a built-in function that returns the data type of some value or “object.” This is the ‘type‘ function and returns the result as a string.
print(type("123")) --Output: string
print(type(123)) --Output: number
print(type(true)) --Output: boolean
print(type(nil)) --Output: nil
print(type(print)) --Output: function
print(type(type)) --Output: function
Because the parenthesis were omitted for print and type, Lua references the function itself rather than attempt to execute them as functions.
Also illustrated here, if a number is enclosed in quotes, it is a string and not a number.
Roblox (not Lua) also provides a function that does a similar thing. The ‘typeof‘ function returns the names of Lua types as well as Roblox specific data types and objects that we will encounter later on.
print(typeof("123")) --Output: string
print(typeof(123)) --Output: number
print(typeof(true)) --Output: boolean
print(typeof(nil)) --Output: nil
print(typeof(print)) --Output: function
print(typeof(type)) --Output: function
print(typeof(typeof)) --Output: function