Let’s create your very first script. In the explorer, highlight the workspace and click on the ‘+.’ Find and click on the ‘Script‘ object.
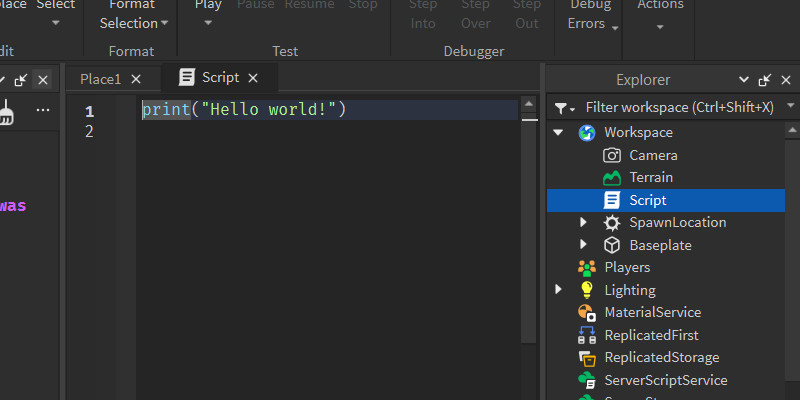
This will add a script into the workspace and open the editor. At the top of the script, you can see that there is already code written to the file:
print("Hello world!")
Press the play button (or F5) to start a game.
Then after your character has loaded into the game, press ‘Stop’ to exit. If you don’t already have the Output window open, enable it from the View tab of the toolbar.
Somewhere in the output window, you should see the phrase ‘Hello world!‘
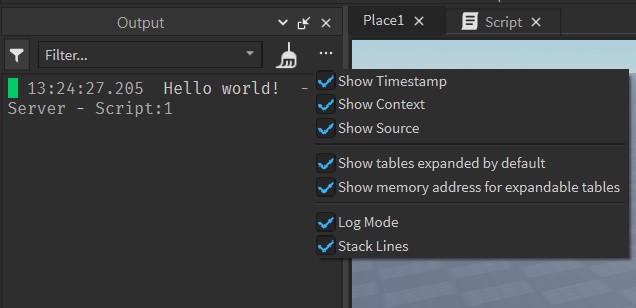
We are only interested in this part of the output so if you click the three dots near the top right of that window, you can disable the other messages.
This pre-written line of code instructs Lua to perform a specific task. We call this a ‘statement.’
As you likely already ascertain, the word ‘print‘ instructs Lua to print the message to the output.
This is a special reserved word and along with the parenthesis form what is known as a ‘function.’
A function is a bit of pre-defined code for performing a specific task. In this case, print something to the output.
Think of a function as a black-box that contains and hides away its inner technical workings. Then every time we want to perform that task again, rather than re-writing all the code, we can just “call” that function.
The parenthesis are what instruct Lua to execute it as a function and passes to that function any data within the parenthesis.
Later on you will learn how to create your own functions.
The ‘Hello world!‘ part is the actual message to be printed. This is what is known as an ‘argument.’
For the print function, it’s possible to give it multiple arguments by separating them with commas:
print("Hello world!", 3.14, 42)
Tip: If you have the Command Bar enabled, you can forgo starting up a play session and just paste code directly into the command bar. This is not useful if you need to test game behavior, but indispensable when you want to quickly run snippets of code.
Comments
Sometimes you might want to include commentary about a section of code or leave out optional code to be toggled later on.
Comments are denoted by double dashes (–). Anytime a line is designated a comment, it gets ignored.
print("Hello world!") --Output: Hello world!
--print("This line is ignored") --Output: [None]
It is also possible to create multiple lines of comments by adding two angle left brackets to open and closed with two right angle brackets:
--[[
print("nothing")
print("happens")
]]
This code has been “commented out.”
You may have noticed that there are also quotes surrounding the message. What is the purpose of the quotes you might ask? Why can’t we just omit the quotes like so?
print(Hello world!)